While list of all Exceptions in Selenium Java itself doesn’t introduce new exceptions, it relies on exceptions from the Java programming language and the WebDriver API. Below are some common exceptions that you may encounter while working with Selenium in Java, along with brief descriptions, reasons, and possible solutions
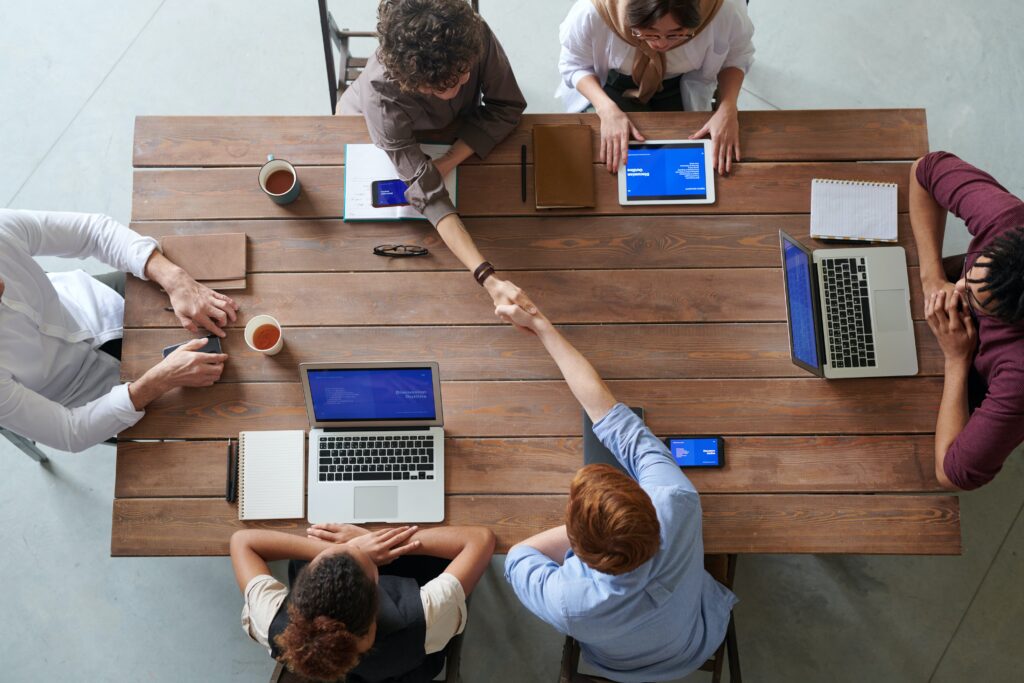
List of all Exceptions in Selenium Java
List of all Exceptions in Selenium Java
Table of Contents
- NoSuchElementException
- Description: Thrown when an element could not be found.
- Reasons: Incorrect locator strategy, element not present in the DOM.
- Solutions: Double-check your locators, use proper waiting strategies like
WebDriverWait
.
- TimeoutException
- Description: Raised when an operation times out.
- Reasons: Implicit or explicit wait timeout.
- Solutions: Increase the timeout duration, optimize your waits, or handle the exception.
- StaleElementReferenceException
- Description: Occurs when a previously found element is no longer valid.
- Reasons: DOM refresh or page navigation.
- Solutions: Re-find the element or navigate back to the page.
- ElementNotVisibleException
- Description: Thrown when an element is present in the DOM but not visible.
- Reasons: Element might be hidden or covered.
- Solutions: Ensure the element is visible before interacting with it.
- ElementNotInteractableException
- Description: Happens when an element is present but not in a state to be interacted with.
- Reasons: Element may be disabled or not ready to accept input.
- Solutions: Wait for the element to become clickable or enabled.
- NoSuchFrameException
- Description: Thrown when switching to a frame that does not exist.
- Reasons: Incorrect frame index or name.
- Solutions: Ensure the frame index or name is correct.
- NoAlertPresentException
- Description: Raised when there is an attempt to switch to an alert that is not present.
- Reasons: No alert on the page.
- Solutions: Handle the exception appropriately or check for alert presence before switching.
- UnexpectedAlertPresentException
- Description: Occurs when an unexpected alert is present.
- Reasons: Unexpected alert popup.
- Solutions: Handle the unexpected alert or dismiss it before continuing.
- ElementClickInterceptedException
- Description: Thrown when a click could not be completed because the element is obscured.
- Reasons: Another element is covering the target element.
- Solutions: Move to the element using
Actions
class or wait for the overlay to disappear.
- ElementNotSelectableException
- Description: Raised when trying to select an unselectable element.
- Reasons: Element might be disabled or not selectable.
- Solutions: Ensure the element is selectable before trying to interact with it.
- InvalidSelectorException
- Description: Thrown when the selector used to find an element is invalid.
- Reasons: Incorrect CSS or XPath selector.
- Solutions: Double-check and correct your selector.
- InvalidElementStateException
- Description: Happens when an element is in an invalid state for an operation.
- Reasons: Element might be disabled or not ready for the requested action.
- Solutions: Ensure the element is in the correct state before performing actions.
- InvalidCookieDomainException
- Description: Raised when trying to add a cookie under a different domain.
- Reasons: Cookie domain mismatch.
- Solutions: Set the cookie domain to match the current domain.
- InvalidCoordinatesException
- Description: Occurs when invalid coordinates are provided.
- Reasons: Incorrect x or y coordinates.
- Solutions: Provide valid coordinates for the action.
- UnhandledAlertException
- Description: Thrown when trying to perform an action while an alert is present.
- Reasons: Attempting an action without handling an existing alert.
- Solutions: Handle the alert before performing the action.
- SessionNotCreatedException
- Description: Raised when a new session could not be created.
- Reasons: WebDriver configuration issue or driver executable not found.
- Solutions: Check WebDriver setup and executable paths.
- UnableToSetCookieException
- Description: Occurs when a cookie cannot be set.
- Reasons: Invalid cookie data.
- Solutions: Provide valid cookie information.
- WebDriverException
- Description: A generic exception for WebDriver-related issues.
- Reasons: Various issues, including network problems or browser crashes.
- Solutions: Investigate the specific cause mentioned in the exception.
- ScreenshotException
- Description: Thrown when there is an issue taking a screenshot.
- Reasons: Insufficient permissions or disk space.
- Solutions: Check permissions and available disk space.
- RemoteDriverServerException
- Description: Raised for issues related to the remote WebDriver server.
- Reasons: Server-side issues.
- Solutions: Check the server logs and ensure the server is running correctly.
- ElementNotDisplayedException
- Description: Occurs when an element is not displayed on the page.
- Reasons: Element might be hidden or not in the viewport.
- Solutions: Ensure the element is visible within the viewport.
- ElementNotEnabledException
- Description: Thrown when trying to interact with a disabled element.
- Reasons: Element is disabled.
- Solutions: Enable the element before performing actions.
- ImeActivationFailedException
- Description: Raised when IME activation fails.
- Reasons: IME activation issues.
- Solutions: Verify IME settings and try again.
- InvalidSessionIdException
- Description: Occurs when an invalid session ID is used.
- Reasons: Expired or invalid session ID.
- Solutions: Refresh or recreate the WebDriver session.
- JavaScriptException
- Description: Thrown for issues related to executing JavaScript.
- Reasons: JavaScript execution error.
- Solutions: Check the JavaScript code for errors.
- MoveTargetOutOfBoundsException
- Description: Raised when the target of a move action is outside the visible area.
- Reasons: Target element is not in the viewport.
- Solutions: Scroll the page or adjust the target coordinates.
- UnhandledPromptException
- Description: Occurs when a user prompt is not handled.
- Reasons: Unexpected user prompt.
- Solutions: Handle the prompt before proceeding.
- UnsupportedCommandException
- Description: Thrown when an unsupported WebDriver command is used.
- Reasons: Unsupported command.
- Solutions: Use supported WebDriver commands.
- WebDriverScriptTimeoutException
- Description: Raised when a script exceeds the maximum execution time.
- Reasons: Long-running script.
- Solutions: Optimize the script or increase the script timeout.
- InvalidElementStateException
- Description: Occurs when the state of an element is not as expected.
- Reasons: Element state mismatch.
- Solutions: Verify the element state before interacting with it.
Remember, handling exceptions appropriately in your Selenium scripts is crucial for robust and stable test automation. Customize your exception handling strategies based on the specific needs of your application and the nature of the exceptions encountered.